Drupal's powerful and flexible architecture allows developers to create complex websites and applications. One key feature that makes Drupal robust is its support for constraints. Constraints are rules that enforce data validation and integrity within your content.
They ensure that the data entered into the system adheres to specific criteria, enhancing the reliability and quality of the content. In this blog post, we'll explore how to work with constraints in Drupal, focusing on custom constraints and how they can be used effectively in your projects.
What are Constraints?
Constraints in Drupal are validation rules applied to data entities. They help ensure that data entered into fields or entities meets certain criteria. Constraints can be applied to:
- Fields (e.g., ensuring an email field contains a valid email address).
- Entities (e.g., ensuring a content type has required fields filled).
- Custom conditions as defined by developers.
Drupal provides several built-in constraints, but you can define custom constraints for more complex scenarios.
Prerequisites
Before you begin, ensure you have:
- A Drupal site is set up and running.
- Administrative access to your Drupal site.
- Basic knowledge of Drupal module development and PHP programming.
Building Constraints
Step 1: Understanding Built-In Constraints
Drupal comes with various built-in constraints that you can apply to your fields and entities. Some common examples include:
- NotBlank: Ensures a field is not empty.
- Email: Validates that the input is a valid email address.
- Length: Restricts the length of the input to a specified range.
These constraints can be easily applied when creating or managing content types and fields through the Drupal administrative interface.
Step 2: Creating Custom Constraints
For more complex validation logic, you may need to create custom constraints. Let's create a custom constraint that ensures a field contains only uppercase letters.
- Create a Custom Module:
First, create a folder for your module in the modules/custom directory, for example, uppercase_constraint. Inside this folder, create an .info.yml file:
2. Define the Constraint:
Create a PHP class for the constraint in src/Plugin/Validation/Constraint/UppercaseConstraint.php:
3. Define the Constraint Validator:
Create the validator class in src/Plugin/Validation/Constraint/UppercaseConstraintValidator.php:
Step 3: Applying the Custom Constraint to a Field / Entity
Now that your custom constraint is defined, you can apply it to a field, entity type or custom entity.
- Adding a Constraint to a Field of an Entity Type
In .module, implement either
hook_entity_base_field_info_alter() (for base fields defined by the entity) or
hook_entity_bundle_field_info_alter() (for fields added to a bundle of the entity):
For fields like title, body, etc use hook_entity_base_field_info_alter()
For other fields than base fields used: hook_entity_bundle_field_info_alter()
- Adding a Constraint to a Base Field of a custom Entity Type
Constraints on base fields are added using BaseFieldDefinition::addConstraint() in overrides of ContentEntityBase::baseFieldDefinitions():
- Adding a Constraint to an Entity Type
In .module, implement hook_entity_type_alter():
- Adding a Constraint to a custom Entity Type
Add the constraint to the entity type annotation on the entity class. If the constraint plugin is configurable, the options can be set there. If it is not, specify an empty array with {}
Step 4: Testing Your Custom Constraint
To ensure your custom constraint works as expected, create or edit a content item of the type you applied the constraint to. Try entering a value in the field that doesn't meet the constraint criteria and verify that the validation error message is displayed.
In the example below, the description field must contain only uppercase letters. If the field is not in uppercase, the validation error message "The Description is not in uppercase." is displayed.
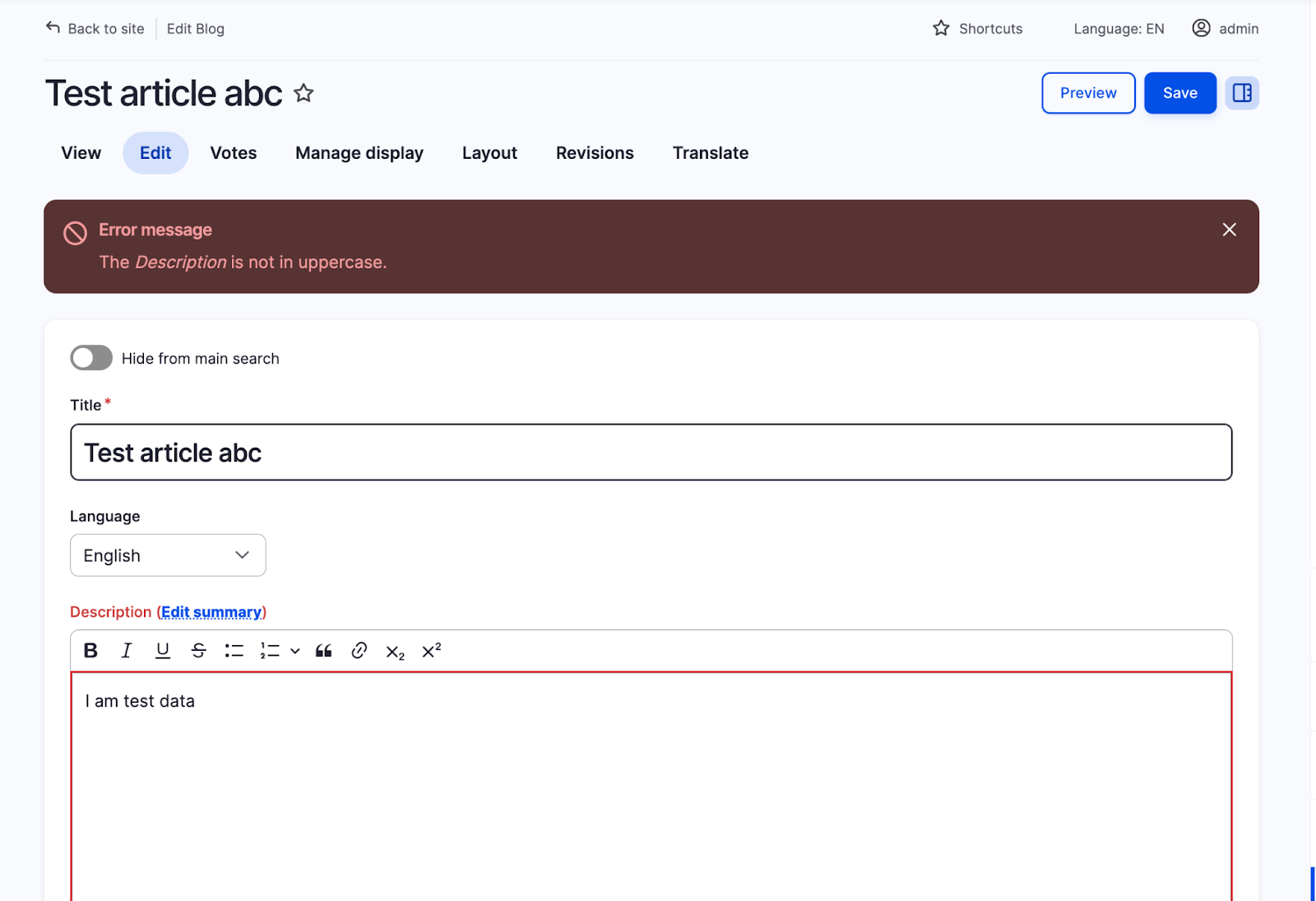
Conclusion
Working with constraints in Drupal helps maintain the integrity and quality of your data. By leveraging built-in constraints and creating custom ones, you can ensure that your content meets specific validation criteria. This guide covered the basics of creating and applying custom constraints in Drupal. With this knowledge, you can build more robust and reliable Drupal applications.
Additional Resources