With the rise of security concerns, traditional username and password systems are often insufficient to protect user data and prevent unauthorized access. Passwords can be easily forgotten, stolen, or hacked, leading to a high risk of breaches, especially in applications handling sensitive or personal information.
Benefits of Implementing an OTP-Based Login Flow in Drupal
This OTP-based login flow is beneficial for users who prefer or require mobile authentication, providing an added layer of security. It reduces the risk of unauthorized access and allows users to bypass traditional password-based login if they wish, which can be especially helpful for those who struggle with password management.
By setting up this flexible, secure OTP verification system, you’re making your Drupal site more secure and appealing, catering to a broader audience that values security and ease of use.
Implementing an OTP-based registration and login system in Drupal addresses several key issues
- Enhanced security
OTP adds a layer of security by requiring users to verify their identity through a code sent directly to their mobile phone. This is known as two-factor authentication (2FA), which greatly reduces the chances of unauthorized access. - Reduced dependence on passwords
Users often struggle with remembering complex passwords, which may lead to weak, reused, or forgotten passwords. OTP systems help minimize this by providing a code that’s valid only for a short duration and used only once. - Improved user experience
For users who have limited access to email or prefer not to receive emails for verification, SMS-based OTP provides a convenient alternative. It's faster, more accessible, and doesn’t require an additional app or service for most users. - Prevention of fake registrations
By requiring a mobile number for verification, this system ensures that users provide valid contact information, helping to filter out fake accounts and spam registrations. - Accessibility for mobile-first users
Many users are more likely to register using their mobile numbers rather than email, especially in regions where mobile internet access surpasses desktop or email usage. OTP-based registration caters to this audience, increasing the accessibility and reach of your application.
In summary, an OTP-based registration and login system in Drupal offers enhanced security, user-friendly registration options, and an improved experience for mobile-first users. Leveraging Twilio and the SMS Framework makes it easy to implement this secure and flexible registration system in Drupal.
Prerequisites
- Twilio SMS framework
Twilio is an API-driven service that can send SMS messages to users, perfect for delivering OTPs. - Drupal modules
Install and configure the SMS Framework and the Twilio modules in Drupal to manage and send SMS messages.
System overview
This solution covers:
- User login/registration
Users can log in or register with an OTP verification process. - Form redirection and validation: Redirect users to a verification form after submitting their mobile number and verify the OTP within a 10-minute window.
- Custom messages: Users receive feedback on whether their code is valid, expired, or incorrect.
User registration flow
To add the "Registration Type" field to the user registration form, you can follow these steps:
- Create the registration type field
- Go to /admin/config/people/accounts/fields.
- Add a new field named "Registration Type".
- Choose List (text) as the field type and label it "Registration Type".
- Configure two options:
- mobile with label "Mobile"
- email with label "Email"
- Save the field.
- Display the field on the registration form
- Once the field is created, go to the Manage form display tab for the user entity.
- Set the "Registration Type" field to be visible on the registration form.
- Custom logic in registration
- In a custom module, use form alter hooks to intercept the registration process:
- Add custom logic to check the "Registration Type" selection.
- If "Email" is selected, proceed with the normal email-based registration.
- If "Mobile" is selected, integrate your OTP logic to send an SMS and redirect to OTP verification.
- If you click on Create account using mobile will create a user account with mobile number and user redirect to otp verification.. If otp verify, user set active
- In a custom module, use form alter hooks to intercept the registration process:
/**
* Form alter for user register form.
*/
function register_user_form_alter(&$form, FormStateInterface $form_state, $form_id) {
if ($form_id == "user_register_form") {
$form['account']['mail']['#states'] = [
'visible' => [
':input[name="registration_type"]' => ['value' => 'email'],
],
];
$form['actions']['submit']['#states'] = [
'visible' => [
':input[name="registration_type"]' => ['value' => 'email'],
],
];
// Add a new submit button create new account using mobile.
$form['actions']['create_account_using_mobile'] = [
'#type' => 'submit',
'#value' => t('Create new account'),
'#submit' => ['register_user_mobile_number_submit'],
'#name' => 'mobile-otp-button',
'#states' => [
'visible' => [
':input[name="registration_type"]' => ['value' => 'mobile_number'],
],
],
];
}
/**
* Custom submit for user register with mobile.
*/
function register_user_mobile_number_submit(array &$form, FormStateInterface $form_state) {
$mobile = $form_state->getValue('field_mobile_number');
if ($mobile) {
$mobile_number = $mobile[0]['value'];
$otp = register_user_generate_login_otp();
// Send otp and verify in the redirected url.
$twilio = \Drupal::service('twilio.sms');
$message = "Your login code is: " . $otp . " Don't share this code with anyone;";
$mobile = '+1' . $mobile_number;
$sid = $twilio->messageSend($mobile, $message);
if ($sid == 'not send') {
\Drupal::messenger()->addError(t('Failed to send SMS.'));
}
else {
// Create a new user.
$user = User::create();
$current_ts = strtotime('now');
// Set the required fields for the new user.
// Use mobile number as username.
$user->setUsername($mobile_number);
// Set the mobile number for email field initially.
$user->set("init", $mobile_number);
$user->set("field_mobile_number", $mobile_number);
$user->set('field_otp_verify', $otp);
$user->set('field_otp_generate_timestamp', $current_ts);
// Save the user.
$user->save();
// Temporary store mobile number to render on otp verify page.
$temp_store_factory = \Drupal::service('tempstore.private');
$temp_store = $temp_store_factory->get('register_user');
$temp_store->set('mobile_number', $mobile_number);
// Redirect to an otp verify form.
$form_state->setRedirect('register_user.user_otp_verify_form');
}
}
}
/**
* Function to generate 6 digit random OTP for user varification.
*/
function register_user_generate_login_otp() {
return str_pad(mt_rand(0, 999999), 6, '0', STR_PAD_LEFT);
}
This setup will add the "Registration Type" field to the registration form and enable conditional handling based on the selected option.

User OTP verification in Drupal
This covers the essential steps to create an OTP verification form in Drupal, with functionality to handle OTP input, validation, and verification using Twilio for SMS.
1. Form setup: UserOtpVerifyForm
- Create a form class to handle OTP verification.
- Define fields for Mobile Number and OTP, with a submission button and a Resend Code option.
2. Form validation
- Ensure the mobile number is in a valid 10-digit format.
- Verify that the OTP is entered.
3. OTP verification logic
- Retrieve the OTP associated with the user's account.
- Check if the OTP matches and is within a 10-minute validity window.
- If valid, activate the user’s account and redirect them to the profile page.
4. OTP resend functionality
- Generate a new OTP and save it to the user's profile along with a timestamp.
- Use Twilio to send the new OTP to the user’s phone.
For full code implementation, check out the GitHub repository.


User login flow
In today’s digital landscape, offering multiple secure authentication options enhances user experience and strengthens security. Implementing an OTP-based login in Drupal is a fantastic way to provide flexibility for users who prefer mobile authentication.
Leveraging Twilio with the SMS Framework, we’ll set up a login workflow that allows users to log in with a mobile number and OTP (One-Time Password). Below, I’ll walk through each component of this setup to outline the OTP-based login flow and provide code snippets to integrate this feature into your Drupal site.
1. Adding an OTP option to the standard login form
The login form starts with the usual Drupal username and password fields but includes an additional link labeled “Login with Mobile & OTP.” When clicked, this link takes users to a form designed specifically for mobile-based login. Here, users have the choice to either continue with the standard method or authenticate via OTP, which is ideal for those who prefer mobile-based access.
/**
* Form alter for user register form.
*/
function register_user_form_alter(&$form, FormStateInterface $form_state, $form_id) {
if ($form_id == 'user_login_form') {
$forgot_password_link = Link::fromTextAndUrl(t('Forgot password?'), Url::fromRoute('user.pass'))->toString();
$mobile_login_link = Link::fromTextAndUrl(t('Use my mobile instead'), Url::fromRoute('register_user.otp_login_form'))->toString();
$form['pass']['#suffix'] = '<div class="form-item forgot-password"><p class="forgot-pass">' . $forgot_password_link . '</p></div>';
$form['actions']['#suffix'] = '<div class="form-item use-mobile"><p class="mobile-login">' . $mobile_login_link . '</p></div>';
}
}

2. Entering the mobile number and sending OTP
On the OTP login form, users are prompted to enter their registered mobile number. After inputting their mobile number, they click “Send OTP.” This triggers a process where an OTP is generated and sent directly to their mobile number via Twilio using the SMS Framework. Once the OTP is sent, the system redirects the user to the OTP verification page to ensure a seamless experience.
Form setup: OtpLoginForm
- Create a form class to handle OTP Login.
- Define fields for Mobile Number with a submission button (Get one-time code.)
- validation for mobile number, also send otp in form submit (Refer git repo for code.)
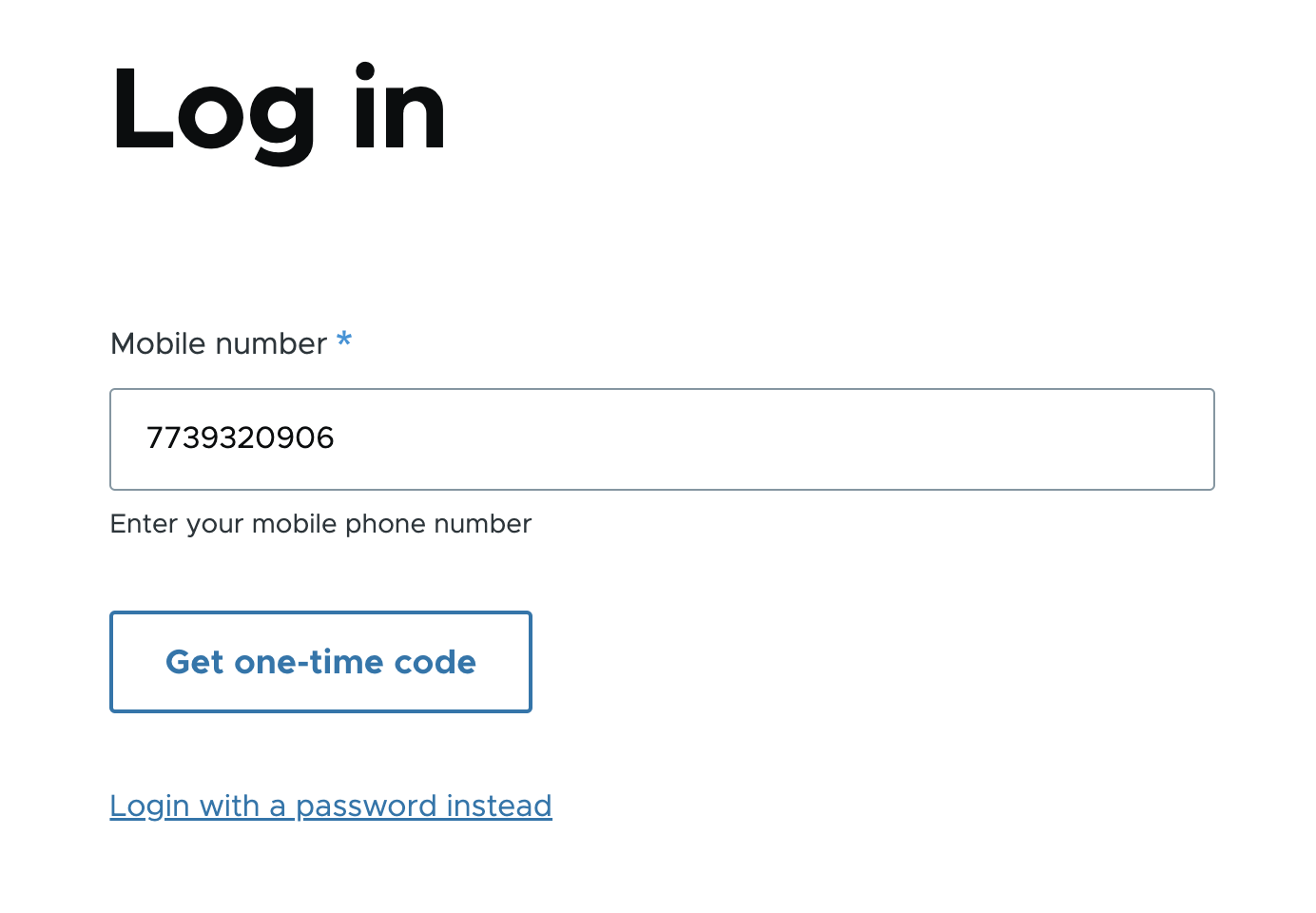
3. OTP verification
On the OTP verification form, users are prompted to enter the OTP they received on their mobile phone. The form validates the OTP and checks if it’s entered within the required time window (typically 10 minutes). If the OTP matches and is within the validity period, the user is logged in and redirected to their account page or a designated landing page.
We have used the same logic that has been used in the user register OTP verification form.
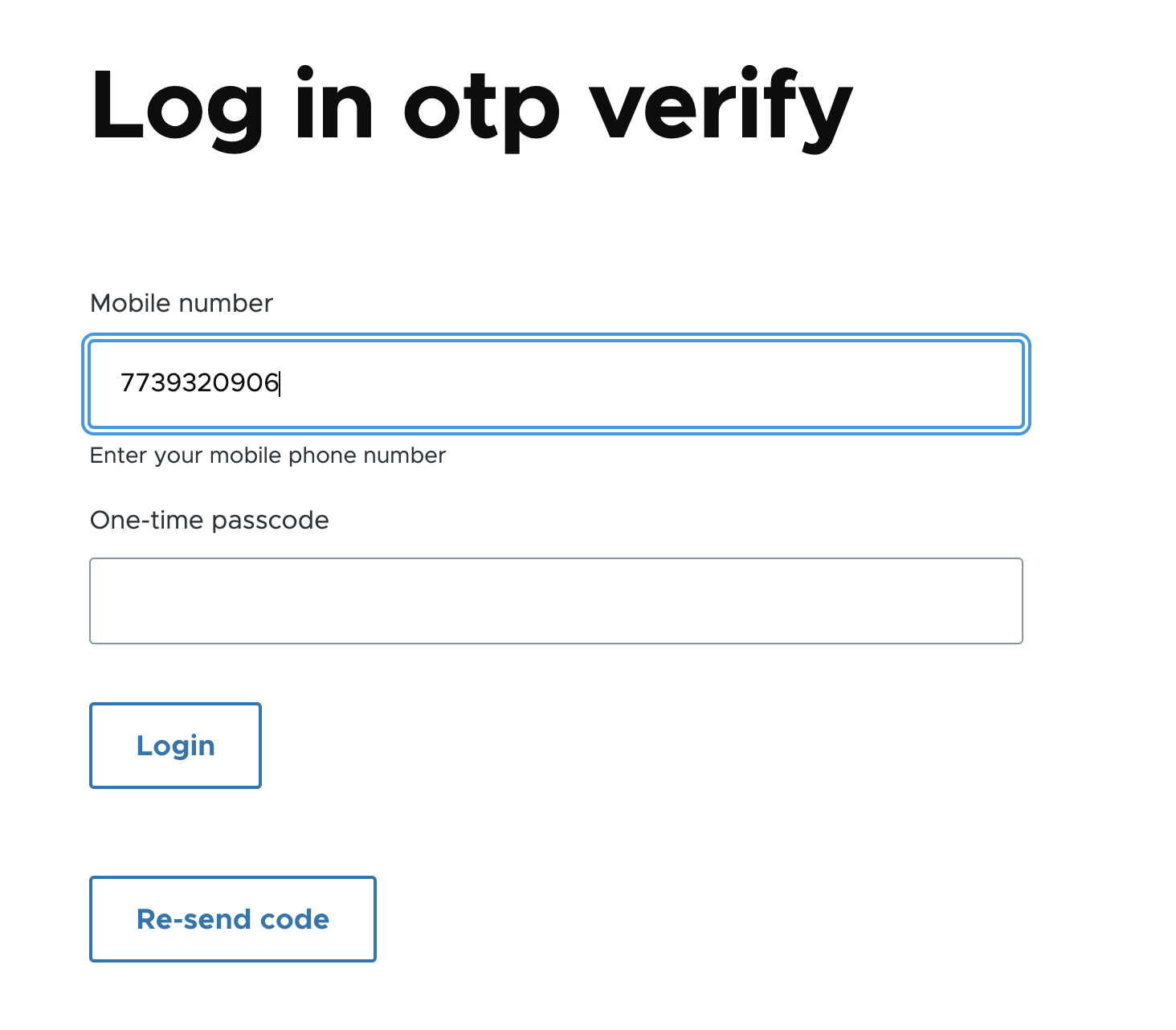
Conclusion
Incorporating Twilio and the SMS Framework into Drupal unlocks the potential for a secure and user-friendly OTP-based login and registration system. This approach not only bolsters security by adding an additional layer of verification but also streamlines the user experience by offering an alternative to traditional password-based methods.
Implementing this system reflects a forward-thinking commitment to both usability and security, catering to the growing demand for seamless, mobile-first solutions. By prioritizing accessibility and protecting against unauthorized access, you enhance trust and engagement among your users.
This guide serves as a foundation to help you develop and customize an OTP verification system tailored to your Drupal site’s unique requirements. The benefits extend beyond immediate security gains, fostering a modern user experience that resonates with today’s mobile-savvy audiences. As digital threats evolve, taking such proactive measures ensures your platform remains relevant, secure, and user-focused in a competitive landscape.