Scaling test automation means building a structured framework that ensures reliability, speed, and maintainability. As applications grow, a well-planned approach keeps test execution efficient, reduces maintenance overhead, and improves confidence in results.
Organizations that invest in structured test automation often achieve 30% faster release cycles and fewer production issues, leading to smoother deployments and better user experiences.
A well-designed Cypress framework supports thousands of test executions while adapting to different environments.
For instance, an e-commerce platform validating payment gateways, user flows, and device compatibility needs a strategy that keeps test execution fast and reliable. Without clear organization, test runs can become time-consuming, delaying releases and increasing operational complexity.
This guide focuses on essential strategies for handling environment configurations, organizing test suites, managing unexpected errors, and optimizing test performance.
With the right approach, it can scale seamlessly, keeping testing efficient and aligned with development goals.
Environment management
A well-planned environment setup keeps test execution smooth across development, staging, and production. Clear environment variables ensure tests run consistently, allowing teams to switch between environments without modifying test files.
This flexibility makes automation scalable and easier to maintain as infrastructure evolves.
To avoid misconfigurations, a fallback mechanism should always be in place. Using clear, easily recognizable environment names helps prevent confusion, ensuring tests run in the right context. Logging the selected environment adds visibility, making it easier to debug issues when running tests under different conditions. A structured approach to environment management keeps testing reliable and efficient, reducing the risk of errors and unexpected failures.
Overview:
Here's how test execution flows across different environments:
- Setting up base URLs
Below is sample code of config.js that is used for Setting up Base URL’s :
Syntax :
// cypress/config/config.js
const environments = {
dev: "https://dev.example.com",
stage: "https://staging.example.com",
prod: "https://www.example.com",
local: "http://localhost:3000"
};
function setBaseUrl() {
const env = process.env.CYPRESS_ENV || "prod";
Cypress.config("baseUrl", environments[env] || environments.prod);
console.log(
`%c🌍 Environment Selected: %c${env}`,
"color: #4CAF50; font-weight: bold",
"color: #2196F3; font-weight: bold"
);
}
Best practices:
- Always include fallback values
- Use descriptive environment names
- Implement clear logging
- Keep sensitive data in environment variables
Shell script automation
Automating test execution makes workflows more efficient by reducing manual steps. A simple shell script can handle environment selection before running Cypress tests, ensuring consistency across different test runs.
This approach is especially useful in CI/CD pipelines, where automation removes the need for manual input and minimizes setup errors.
To keep things reliable, the script should validate inputs and allow only predefined environments. Assigning a default environment helps prevent issues caused by missing configurations. Adding clear logs makes it easy to see which environment is selected, giving teams instant feedback and helping them troubleshoot quickly when needed.
- Script setup
First, create a shell script for test execution:
Below is sample code of setTestEnv.sh that is used to select environment :
Syntax:
// setTestEnv.sh
#!/bin/bash
print_log() {
COLOR=$1
MESSAGE=$2
echo -e "\033[0;${COLOR}m${MESSAGE}\033[0m"
}
get_environment() {
while true; do
read -p "Enter environment (prod/dev/stage/local) [default: prod]: " ENV
ENV=${ENV:-prod}
case $ENV in
prod|dev|stage|local) break ;;
*) print_log "red" "Invalid environment. Please try again." ;;
esac
done
}
- Implementation steps
- Create the script file
- Add environment selection
- Implement test path configuration
- Add validation and error handling
Flowchart :
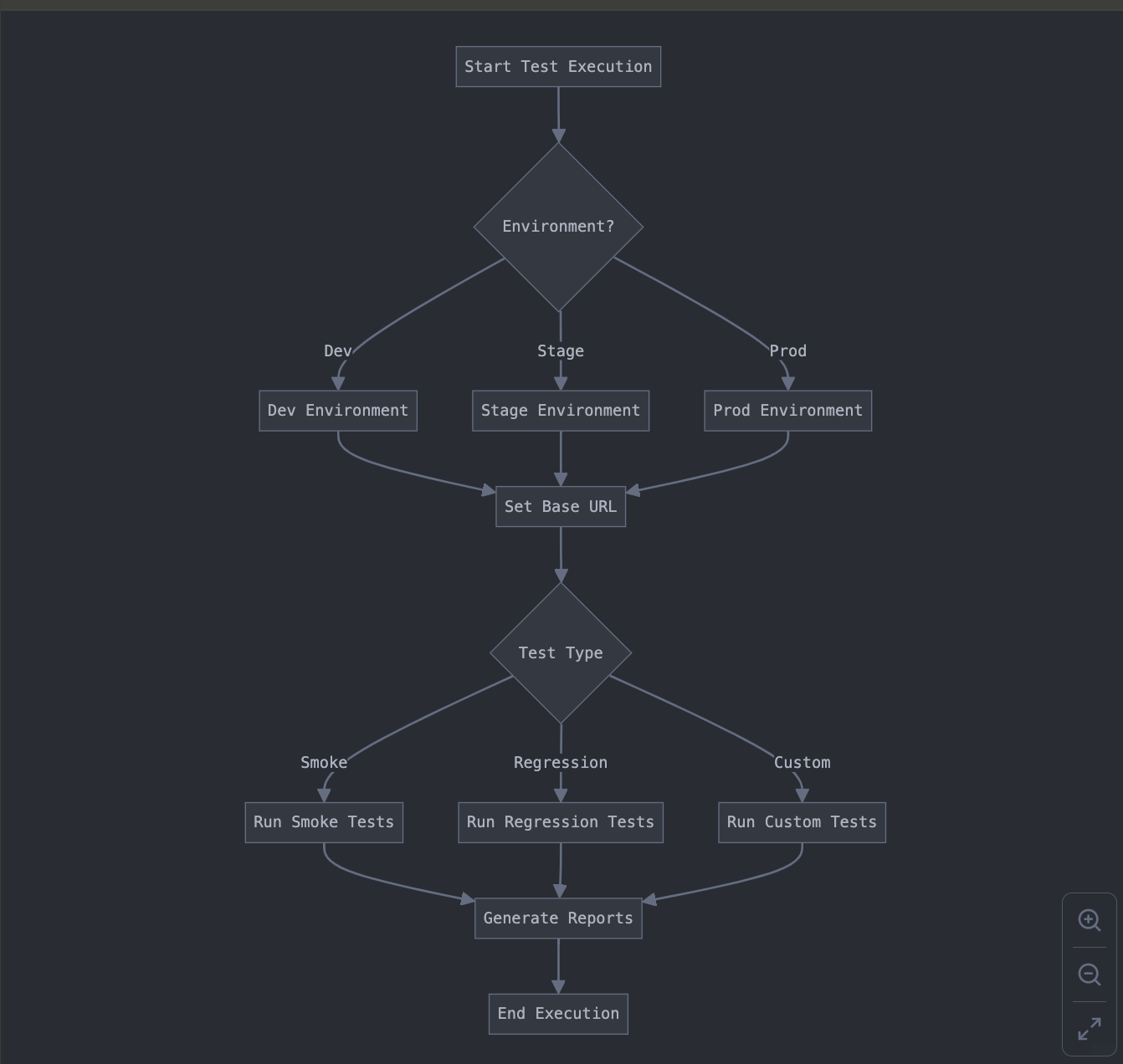
Shell script automation flowchart
Test organization and filtering
A well-structured test suite makes test execution more efficient and easier to manage. When tests are organized by priority, module, and feature, teams can run only what’s necessary instead of executing the entire suite every time. This keeps test runs faster and more relevant to current development needs.
Using test tags like ‘smoke,’ ‘regression,’ or ‘high-priority’ helps teams filter and execute specific test groups, making workflows more streamlined. Well-tagged tests are easier to maintain and adapt as the application evolves.
Clear naming conventions for test files and folders also play a key role in keeping the repository structured. When test cases follow a logical order, team members—especially new ones—can navigate the framework effortlessly without confusion. A well-organized test suite not only saves time but also makes scaling automation much more manageable.
- Test suite organization
Here's a recommended structure for organizing your tests:
- Setting up test tags
Below is sample code of Setting test tags that is used to select and execute specific test groups like smoke sanity and based on the priorities :
Syntax :
// File : TestLogin.cy.js
describe('Login Tests', () => {
it('should handle login process', {
tags: ['smoke', 'auth', 'high']
}, () => {
// Test implementation
});
});
describe('PIM Tests', () => {
it('should create new product', {
tags: ['smoke', 'pim', 'high']
}, () => {
// Test implementation
});
});
- Tag categories
- Priority: high, medium, low
- Type: smoke, sanity, regression
- Module: pim, marketo, orders
- Feature: login, search, checkout
Flowchart :

Test organization and filtering flowchart
Error handling
A reliable automation framework needs to handle unexpected errors effectively. Issues like network delays, dynamic UI changes, and unhandled exceptions can affect test stability. A strong error-handling approach helps keep test runs consistent and prevents false failures from slowing down development.
Cypress offers built-in hooks to catch uncaught exceptions, log useful details, and keep tests running without unnecessary terminations. Clear logging makes it easier to diagnose issues, and setting limits on exception handling ensures that frequent failures don’t mask deeper problems that need attention.
Writing tests with real-world variations in mind improves reliability. Dynamic elements, API response delays, and UI updates should be accounted for to prevent flaky tests. A well-structured error management strategy creates a stable automation framework, reducing test failures and keeping execution smooth.
- Error handling flow
Here's how different types of errors are managed:ImplementationBelow is sample code of Handle Uncaught Exceptions that is used to solve exceptions and console errors while loading the webpage : Create File : error-handler.js
Syntax :
// cypress/support/error-handler.js
const handleUncaughtExceptions = () => {
let uncaughtExceptionCount = 0;
Cypress.on("uncaught:exception", (err, runnable) => {
uncaughtExceptionCount++;
console.error(`Uncaught Exception #${uncaughtExceptionCount}:`, {
message: err.message,
stack: err.stack,
testTitle: runnable.title
});
if (uncaughtExceptionCount > 5) {
return true;
}
return false;
});
};
Flowchart :
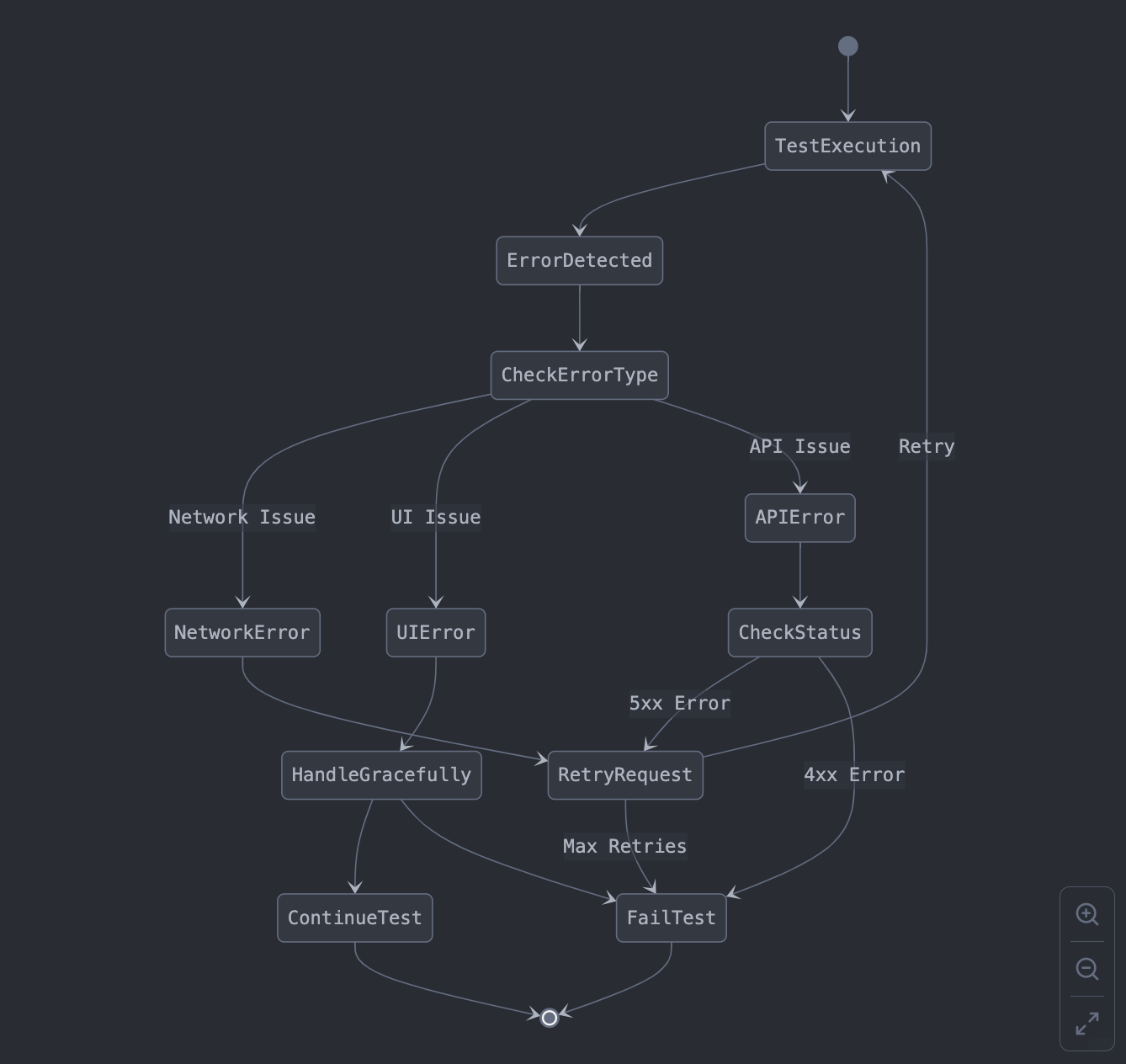
Error handling flowchart
Feature flag management
Feature flags help test automation stay in sync with evolving application functionalities. When new features roll out gradually, tests should adjust dynamically to account for their availability. By using environment variables, cypress can check whether a feature is enabled and decide whether to test the latest version or stick with the existing implementation.
This approach keeps test runs stable, avoiding unnecessary failures when a feature is still in development. It also supports A/B testing by allowing different test scenarios without disrupting the overall suite.
Integrating feature flags into Cypress ensures tests stay relevant as the application grows, keeping automation flexible and aligned with real-world usage.
Implementation
Syntax :
describe('Login Tests', () => {
let useLatestFeature;
beforeEach(() => {
const LFValue = Cypress.env('LF');
useLatestFeature = LFValue === 'true' || LFValue === true;
});
it('should handle login process', {
tags: ['smoke', 'auth']
}, () => {
if (useLatestFeature) {
loginDirect();
} else {
loginLegacy();
}
});
});
Performance optimization
Efficient test execution is essential for large-scale applications. Optimizing test runs ensures faster execution without sacrificing coverage. Using precise selectors improves stability and speeds up test execution, while avoiding generic or unreliable locators reduces flakiness.
Event-based synchronization ensures that tests proceed only when the application is ready, improving consistency.
Managing resources effectively helps maintain performance. Running too many parallel tests can strain system memory, slowing down execution rather than improving it. A balanced approach—limiting parallel execution based on available resources—prevents unnecessary slowdowns.
Test data should also be structured to avoid dependencies that can cause unpredictable failures.
Data-driven testing helps reduce redundancy by covering multiple scenarios with fewer test cases. Regularly reviewing and refining test cases keeps the suite focused, eliminating outdated or overlapping tests that add unnecessary overhead.
Best practices for optimized execution
- Use precise selectors to improve stability and execution speed.
- Replace arbitrary wait times with event-based synchronization for consistency.
- Manage resources by optimizing parallel test execution to prevent performance bottlenecks.
- Implement data-driven testing to maintain coverage without redundant test cases.
- Continuously review and refine test cases to keep the suite efficient.
A well-optimized test execution strategy ensures that Cypress tests remain fast, reliable, and scalable, supporting smooth development and deployment cycles.
Ensuring viewport management
Applications today run across multiple devices, from smartphones to large desktop screens. Testing needs to account for these variations to ensure a consistent user experience.
A well-planned viewport management strategy helps validate how applications respond to different screen sizes, making sure layouts, navigation, and functionality remain intact across mobile, tablet, and desktop resolutions.
Rather than relying on default settings, defining viewport configurations for specific devices ensures accurate testing. Applying these presets consistently across test cases helps catch display issues early and prevents inconsistencies.
Responsive testing should reflect how real users interact with the application, ensuring that buttons, menus, and key features remain accessible and functional at every screen size. Proper viewport management helps prevent layout shifts, overlapping elements, or navigation problems that could otherwise surface in production.
- Viewport presets
Syntax :
const viewports = {
mobile: { width: 375, height: 667 },
tablet: { width: 768, height: 1024 },
desktop: { width: 1920, height: 1080 }
};
// Usage in tests
describe('Responsive Testing', () => {
it('works on mobile', () => {
cy.viewport(viewports.mobile.width, viewports.mobile.height);
// Test implementation
});
});
Flowchart :
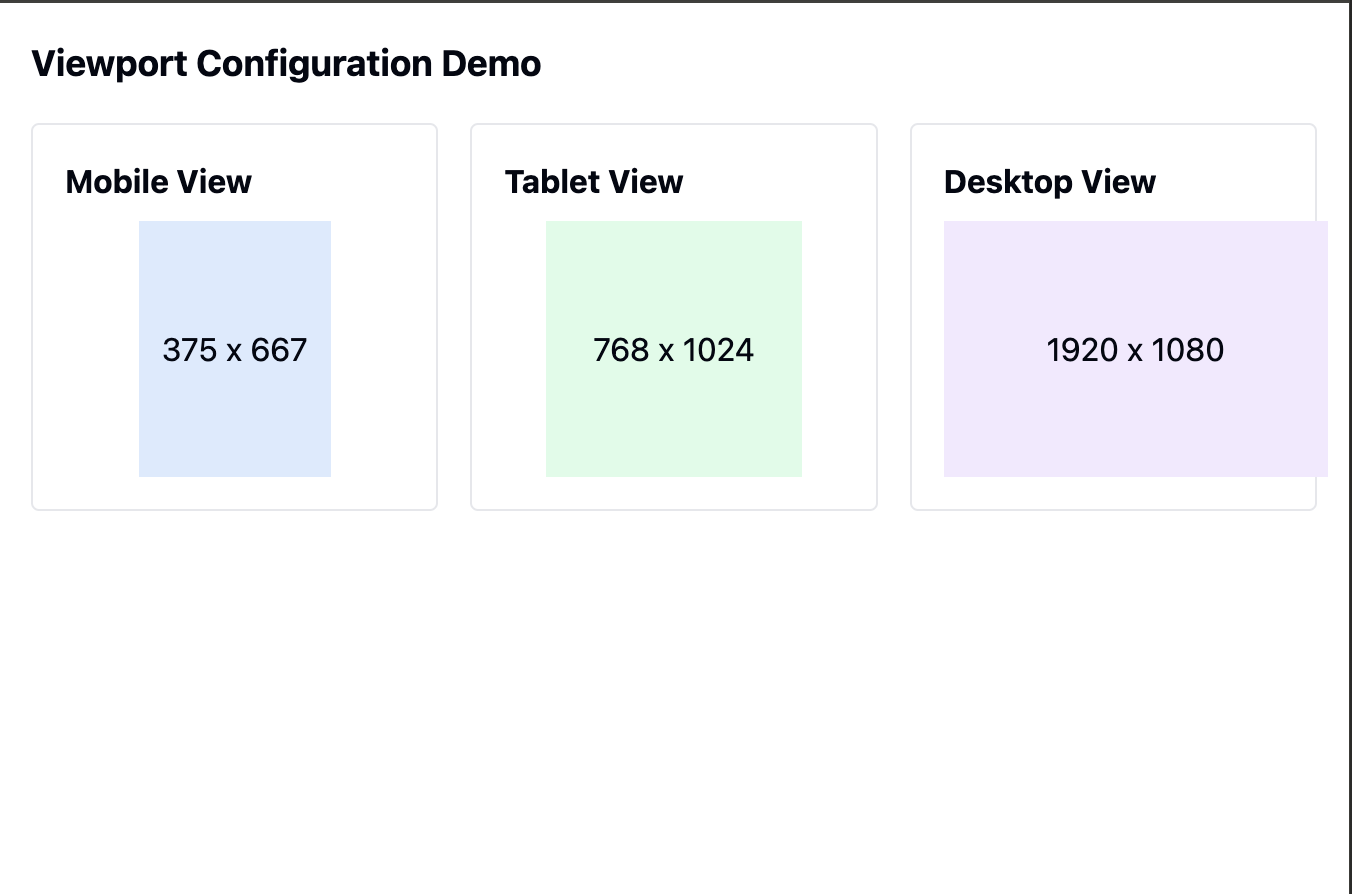
Virewport configuration demo
Reporting and screenshots for better debugging
Clear reporting makes it easier to track test results and troubleshoot failures efficiently.
Cypress offers various reporting tools that generate detailed execution reports, complete with charts, logs, and embedded screenshots. These reports help teams quickly analyze outcomes, spot patterns, and address issues before they impact production.
Screenshots captured during test failures provide instant visual context, making debugging faster and more precise. Video recordings add another layer of insight by showing the exact sequence of interactions leading up to an issue.
With well-structured reporting, developers, testers, and stakeholders can collaborate more effectively, ensuring test results are transparent and actionable.Setup
Syntax :
// cypress.config.js
module.exports = {
reporter: 'cypress-mochawesome-reporter',
video: true,
screenshotOnRunFailure: true,
reporterOptions: {
charts: true,
reportPageTitle: 'Test Execution Report',
embeddedScreenshots: true
}
};
Leveraging latest cypress features
Staying updated with Cypress improvements enhances test capabilities.
Features like component testing allow validation of UI elements in isolation, ensuring they function correctly before integration.
Newer Cypress versions include performance optimizations, improved debugging options, and better support for modern frameworks.
Keeping up with updates helps teams leverage improvements that enhance test reliability and execution efficiency.Component Testing// Example React component test
Syntax :
import MyComponent from './MyComponent'
describe('MyComponent.cy.js', () => {
it('renders correctly', () => {
cy.mount(<MyComponent />)
cy.get('[data-testid="my-component"]').should('be.visible')
});
});
Conclusion
"The best test suite is one that confirms quality before users even notice—and remains effortless to maintain."
A well-structured Cypress automation framework makes testing easier to scale. With a clear environment setup, organized test structures, and strong error handling, teams can build a system that is both reliable and easy to maintain. Beyond just writing tests, a well-planned approach ensures that automation keeps up with development, providing fast feedback and supporting continuous improvement.
In my experience, automation works best when it’s designed for long-term efficiency. A defined environment configuration keeps execution smooth across different stages, while automation scripts reduce manual effort and allow teams to focus on development. Well-organized test suites make it easy to run specific test sets when needed, adapting to different priorities without unnecessary overhead.
Handling errors effectively strengthens test stability and ensures consistent results. Tests that account for real-world scenarios provide meaningful insights, making automation a valuable asset rather than just a verification tool. Feature flags make testing even more adaptable, allowing new functionality to be validated without disrupting workflows.
Optimizing execution improves both speed and coverage. Small refinements—such as using precise selectors and event-driven waits—help keep tests efficient without sacrificing reliability. Responsive testing ensures applications perform well across devices, while structured reporting simplifies debugging and provides clear insights for teams.
A strong automation foundation keeps testing efficient and aligned with evolving needs. Structured configurations, automated workflows, and well-organized test cases make everything more manageable. With early error handling, feature flags, and ongoing optimizations, test automation stays scalable and adaptable over time.
Testing supports progress by providing confidence in every release.