Getting your website noticed is no small feat, especially when competing with millions of others online. Search Engine Optimization (SEO) is what makes your site easier to find, helping it rank higher in search results and bring in more visitors.
Imagine you're building an online store with Next.js 14+ and TypeScript. You want customers to find your products when they search for them, and you also want your site to load fast and feel smooth.
This is where Next.js and TypeScript come in. With features like server-side rendering (SSR), dynamic routing, and automatic image optimization, Next.js helps you create websites that search engines love.
In this guide, we'll look at practical ways to boost SEO with Next.js and TypeScript. You’ll learn how to use SSR to deliver content faster, manage metadata to improve your page rankings, and optimize images so they load quickly without sacrificing quality. We'll also cover how dynamic routing can make your URLs more user-friendly, which is great for both search engines and visitors.
Whether you’re building a small personal blog or a feature-packed e-commerce site, these techniques will help you create a website that ranks higher, works better, and keeps visitors coming back.
Let’s dive into how you can combine the latest tools and techniques to make your website a success.
What is SEO ?
Search Engine Optimization, or SEO, is about making your website easier for search engines like Google to find and understand.
When your site is optimized for search engines, it has a better chance of showing up higher in search results when people look for topics related to your content. This can help your website attract more visitors, grow its audience, and achieve its purpose.
Think of search engines as librarians for the internet. When someone searches for something, the search engine scans its vast library of websites to find the best matches.
SEO helps your website stand out to these search engines by showing them that your site is trustworthy, relevant, and easy to navigate.
Next.js makes it easier to build SEO-friendly websites by providing tools like:
- Server-Side Rendering (SSR): This ensures that your webpage is fully prepared when a search engine looks at it, including all its content. This helps search engines understand your site better and improves its chances of ranking higher.
- Static Site Generation (SSG): This creates fast and pre-built pages that load instantly, offering a great user experience while being friendly to search engines.
- Dynamic Routing: This allows your website to create clean and descriptive web addresses (URLs), which both users and search engines appreciate.
Setting Up a Next.js App with TypeScript
The initial step is to create a Next.js app configured with TypeScript. You can do this by running the following command:
npx create-next-app@latest seo-nextjs — typescript
This will set up a new Next.js project preconfigured for TypeScript. For more details, visit the Next.js documentation.
SEO Friendly URLs
A well-structured website URL hierarchy can benefit our websites ranking. Clean and descriptive URLs improve both user experience and SEO. Next.js enables the creation of dynamic routes, making it simple to build SEO-friendly URLs.
Next.js uses file-system routing built on the concept of pages. When a file is added to the pages directory, it is automatically available as a route. The files and folders inside the pages directory can be used to define most common patterns.
Let’s take a look at a couple of simple URLs :
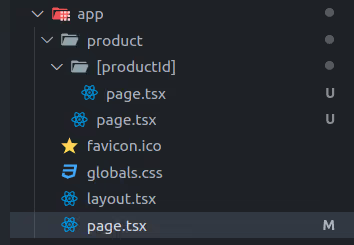
Homepage: https://www.example.com → app/page.tsx
- This serves as the homepage of your application.
- URL corresponds directly to /.
Listings: https://www.example.com/product → app/product/page.tsx
- This defines the route for the product listings page.
- URL corresponds directly to /product.
Detail: https://www.example.com/product/1 → app/product/[productId]/page.tsx
- This dynamic segment [productId] allows for detail pages for each product.
- URL corresponds to /product/:productId (e.g., /product/1 or /product/42).
Optimizing Images and Multimedia
Image optimization is a fundamental SEO factor. With Next.js, we can use the next/image component to ensure responsive image loading and efficient optimization. Don’t forget to provide descriptive alt attributes for our images to improve accessibility and SEO.
import Image from 'next/image';
export default function ImageComponent() {
return (
<Image
src='/img.png'
width={500}
height={500}
alt='alt-text'
/>
);
}
XML Sitemaps
XML sitemaps provide search engines with a map of our website’s structure, making it easier for them to index our content. The next-sitemap package simplifies the generation of XML sitemaps.
Here’s an example where we generate sitemap entries for a homepage, a product listing page, and individual product pages dynamically fetched from an API:
import type { MetadataRoute } from 'next'
const getProducts = () => {... // Fetch and return Products
}
export default function sitemap(): MetadataRoute.Sitemap {
type SitemapEntry = {
url: string;
lastModified?: string | Date;
changeFrequency?: 'daily' | 'yearly' | 'always' | 'hourly' | 'weekly' | 'monthly' | 'never';
priority?: number;
};
const baseUrl = 'https://www.example.com';
const productUrl = '/product';
const productsRes = getProducts(); // Fetch products from an API
const productsData: SitemapEntry[] = productsRes?.map((productEle) => ({ // Map products to sitemap format
url: `${baseUrl}${productUrl}/${productEle?.slug}`,
lastModified: productEle?.createdDate || new Date(),
changeFrequency: 'daily',
priority: 1,
})) || [];
const sitemap: SitemapEntry[] = [ // Combine static and dynamic entries
{
url: baseUrl, // Homepage
lastModified: new Date(),
changeFrequency: 'yearly',
priority: 1,
},
{
url: `${baseUrl}${productUrl}`, // Product Listing page
lastModified: new Date(),
changeFrequency: 'monthly',
priority: 1,
},
...productsData, // Product Detail Pages
];
return sitemap;
}
Define Static URLs
- The homepage (baseUrl) and product listing page (${baseUrl}${productUrl}) are defined as static entries in the sitemap.
- These URLs are included with their respective changeFrequency and priority settings to guide search engines.
Fetch Dynamic Data
- Use an API call to fetch the list of products. Each product’s slug is used to construct its unique URL.
- The lastModified field ensures search engines know the last time the resource was updated.
Map Dynamic Data to Sitemap Format
- For each product, we create an object containing url, lastModified, changeFrequency, and priority.
- The changeFrequency field indicates how often the content is likely to change, helping search engines prioritize crawling.
Combine Static and Dynamic Entries
- The static entries are merged with the dynamic product entries to form the complete sitemap.
Generated XML Sitemap

Robots.txt
In Next.js, a robots.txt file is used to provide instructions to search engine crawlers (e.g., Googlebot, Bingbot) about which pages or sections of your site should be indexed or ignored. It helps manage search engine visibility for your website.
- userAgent: Defines which crawlers the rules apply to. Use * to apply the rules universally.
- allow: Specifies paths that crawlers are permitted to access.
- disallow: Blocks access to paths you don’t want crawled (e.g., /private/).
- sitemap: Points to the sitemap URL, helping crawlers discover and index your pages efficiently.
Here’s an example how to configure a robots.txt file in a Next.js app using the MetadataRoute.Robots type provided by Next.js
import type { MetadataRoute } from 'next'
export default function robots(): MetadataRoute.Robots {
const baseUrl = 'https://www.example.com';
return {
rules: {
userAgent: '*',
allow: ['/', '/product'],
disallow: '/private/',
},
sitemap: `${baseUrl}/sitemap.xml`,
}
}
Generated Robots.txt

SEO Metadata
The Metadata API in Next.js simplifies defining metadata for your application, such as meta tags and link tags, inside the HTML <head> element. This helps improve SEO and makes your pages more shareable on social media platforms.
You can export either:
- A static metadata object for predefined metadata.
- A dynamic generateMetadata function for generating metadata dynamically based on runtime data.
These exports go in layout.js or page.js files associated with your routes.
Static Metadata
Static metadata in Next.js refers to predefined, unchanging metadata values added to your application for SEO and social sharing purposes. These metadata values, such as titles, descriptions, and Open Graph tags, are set at build time and do not change dynamically based on runtime conditions.
Here’s an example of static metadata defined in a layout file in a Next.js application. It provides default metadata for the pages within the layout, ensuring consistency across the section of the app.
import type { Metadata } from "next";
import "./globals.css";
export const metadata: Metadata = {
metadataBase: new URL('https://www.example.com'),
title: {
default: 'Example',
template: `%s | Example`,
},
keywords: ['keyword1', 'keyword2', 'keyword3'],
openGraph: {
description: 'This is a meta description...',
images: [''],
},
};
export default function RootLayout({
children,
}: Readonly<{
children: React.ReactNode;
}>) {
return (
<html lang="en">
<body>
{children}
</body>
</html>
);
}
metadataBase
- Specifies the base URL of the website.
title
- default: The default title of the page when no specific title is set.
- template: A string template for generating titles dynamically.
keywords
- An array of keywords for SEO purposes.
openGraph
Contains metadata used for Open Graph protocol, which enhances link previews on platforms like Facebook and LinkedIn.
- description: A brief description of the page.
- images: An array of URLs pointing to images used for link previews.
Generated Meta tags in DOM

Dynamic Metadata
Dynamic metadata in Next.js allows you to programmatically generate and customize metadata values based on runtime data, such as page content, user preferences, or external APIs. Unlike static metadata, which is predefined and consistent across pages, dynamic metadata can change depending on the context, providing a more tailored experience for each page.
Dynamic metadata is especially useful for pages that rely on user input or content that changes frequently, such as blog posts, product details, or user profiles.
Here’s an example of dynamic metadata defined in a product file in a Next.js application. It provides dynamic metadata for the product detail pages based on data fetched from API.
import React from 'react';
import { notFound } from 'next/navigation';
export async function generateMetadata({ params }: { params: { productId: string } }){
try{
// read route params
const id = params?.productId;
// fetch product data
const response = await fetch(`https://.../${id}`).then((res) => res.json());
if(response?.length === 0){
return{
title: 'Not Found',
description: 'The page you are looking for does not exist'
}
}
return {
openGraph: {
title: response[0].title,
description: response[0].description,
images: [''],
},
};
} catch(error){
console.error(error);
return{
title: 'Not Found',
description: 'The page you are looking for does not exist'
}
}
}
const page = ({ params }: { params: { productId: string } }) => {
if (parseInt(params?.productId) > 5) {
notFound();
}
return (
<div>HTML code hoes here</div>
);
};
export default page;
Extract Route Parameters: The productId is extracted from params.
Fetch Product Data: Fetches data from API for the product using the productId.
Returns Dynamic Metadata:
If the product is not found (response?.length === 0), a fallback metadata object is returned with a “Not Found” title and description.
If the product is found, metadata is populated using the product data.
Error Handling: If any error occurs during the process, a fallback metadata object is returned.
Conclusion
SEO is essential for making your website visible to the right audience, and with the capabilities of Next.js 14+ and TypeScript, building an SEO-friendly web application has never been more straightforward.
From server-side rendering and static site generation to optimized images, dynamic routes, and detailed metadata management, Next.js provides all the tools you need to create high-performing websites that search engines love.
By combining these features with a thoughtful approach to your website’s structure, content, and accessibility, you can enhance your search engine rankings while delivering a seamless user experience.
Whether you’re crafting a small project or managing a large-scale application, leveraging the power of Next.js and TypeScript ensures your site is ready to meet modern SEO demands effectively.